Introduction to JavaScript Switch Statements
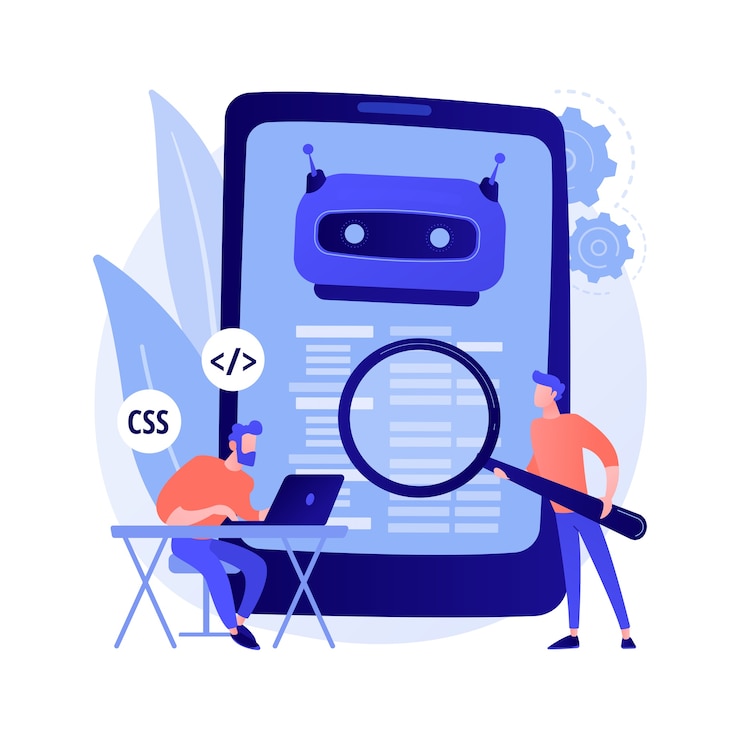
In JavaScript, the switch statement provides a way to execute different blocks of code based on the value of a variable. It allows you to compare a single variable against multiple values and perform different actions depending on the match. In this article, we will introduce switch statements in JavaScript and provide examples to illustrate their usage.
Syntax of Switch Statement
The basic syntax of a switch statement in JavaScript is as follows:
switch (expression) {
case value1:
// Code to be executed if expression matches value1
break;
case value2:
// Code to be executed if expression matches value2
break;
default:
// Code to be executed if expression does not match any values
break;
}
Example:
Consider the following example that determines the day of the week based on a numeric value:
var day = 3;
var dayName;
switch (day) {
case 1:
dayName = "Sunday";
break;
case 2:
dayName = "Monday";
break;
case 3:
dayName = "Tuesday";
break;
case 4:
dayName = "Wednesday";
break;
case 5:
dayName = "Thursday";
break;
case 6:
dayName = "Friday";
break;
case 7:
dayName = "Saturday";
break;
default:
dayName = "Invalid day";
break;
}
console.log("Day: " + dayName);
JavaScript Switch Statement with Fallthrough
In JavaScript, the switch statement allows for fallthrough behavior, which means that if a case matches, the code execution will continue to the next case unless a break
statement is encountered. This feature provides flexibility when multiple cases share the same code block. In this article, we will explore the usage of fallthrough in switch statements with examples.
Example:
Consider the following example that determines the season based on a month value:
var month = 6;
var season;
switch (month) {
case 12:
case 1:
case 2:
season = "Winter";
break;
case 3:
case 4:
case 5:
season = "Spring";
break;
case 6:
case 7:
case 8:
season = "Summer";
break;
case 9:
case 10:
case 11:
season = "Fall";
break;
default:
season = "Invalid month";
break;
}
console.log("Season: " + season);
JavaScript Switch Statement with Default Case
In JavaScript, the switch statement allows you to specify a default case that will be executed if none of the cases match the given expression. The default case provides a fallback option when none of the expected values are found. In this article, we will explore the usage of the default case in switch statements with examples.
Example:
Consider the following example that determines the price of a product based on its category:
var category = "Electronics";
var price;
switch (category) {
case "Books":
price = 10;
break;
case "Electronics":
price = 100;
break;
case "Clothing":
price = 50;
break;
default:
price = "Price not available";
break;
}
console.log("Price: " + price);
JavaScript Switch Statement with Multiple Cases
In JavaScript, the switch statement allows for multiple cases to share the same code block. This feature allows you to handle different values with a common action, reducing code duplication. In this article, we will explore the usage of multiple cases in switch statements with examples.
Example:
Consider the following example that determines the shipping cost based on the weight of a package:
var weight = 3;
var shippingCost;
switch (weight) {
case 1:
case 2:
case 3:
shippingCost = 10;
break;
case 4:
case 5:
case 6:
shippingCost = 15;
break;
case 7:
case 8:
case 9:
shippingCost = 20;
break;
default:
shippingCost = "Shipping cost not available";
break;
}
console.log("Shipping Cost: $" + shippingCost);
0 Comments