Introduction to the JavaScript typeof Operator
The `typeof` operator is a built-in operator in JavaScript that allows you to determine the data type of a value or expression. It returns a string indicating the type of the operand. In this article, we will introduce the `typeof` operator in JavaScript and provide examples to illustrate its usage.
Usage of the `typeof` Operator
The `typeof` operator can be used in the following ways:
- With a variable or constant:
typeof variable
- With a literal value:
typeof value
- With an expression:
typeof expression
Example:
Consider the following example that demonstrates the usage of the `typeof` operator:
var name = "John";
var age = 30;
var isMarried = false;
console.log(typeof name); // "string"
console.log(typeof age); // "number"
console.log(typeof isMarried); // "boolean"
Data Types and the JavaScript typeof Operator
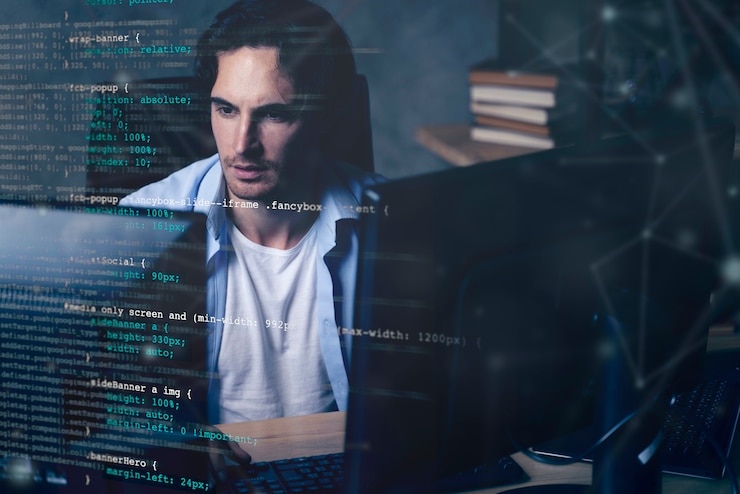
JavaScript has several built-in data types, and the `typeof` operator can be used to determine the type of a value or expression. It helps in performing type-checking operations and handling values based on their data types. In this article, we will explore the relationship between data types and the `typeof` operator in JavaScript with examples.
Common Data Types in JavaScript
JavaScript has the following commonly used data types:
- String: Represents textual data
- Number: Represents numeric data
- Boolean: Represents true/false values
- Object: Represents a collection of key-value pairs
- Undefined: Represents an uninitialized variable
- Null: Represents the absence of any object value
Example:
Consider the following example that demonstrates the usage of the `typeof` operator with different data types:
var name = "John";
var age = 30;
var isMarried = false;
var person = { name: "John", age: 30 };
var car;
var nothing = null;
console.log(typeof name); // "string"
console.log(typeof age); // "number"
console.log(typeof isMarried); // "boolean"
console.log(typeof person); // "object"
console.log(typeof car); // "undefined"
console.log(typeof nothing); // "object" (Note: typeof null is "object" due to historical reasons)
Advanced Usage of the JavaScript typeof Operator
The `typeof` operator can be used in various advanced scenarios in JavaScript. It is commonly used for type-checking, validating function arguments, and handling different types of values. In this article, we will explore the advanced usage of the `typeof` operator in JavaScript with examples.
Type-Checking with `typeof`
The `typeof` operator is often used for type-checking operations. It allows you to determine the data type of a value and perform appropriate actions based on the type. For example:
function greet(name) {
if (typeof name === "string") {
console.log("Hello, " + name);
} else {
console.log("Invalid input!");
}
}
greet("John"); // "Hello, John"
greet(123); // "Invalid input!"
Handling Undefined Values
The `typeof` operator can be used to handle undefined values. It allows you to check if a variable or property is undefined before using it. For example:
var name;
var age = 30;
if (typeof name !== "undefined") {
console.log("Name: " + name);
} else {
console.log("Name is undefined");
}
console.log("Age: " + age);
Using the JavaScript typeof Operator in Conditionals
The `typeof` operator can be used in conditionals to check the data type of a value or expression and execute different code blocks based on the type. It provides flexibility in handling different data types and performing appropriate actions. In this article, we will explore the usage of the `typeof` operator in conditionals in JavaScript with examples.
Conditional Statements based on Data Types
You can use the `typeof` operator in conditional statements to perform actions based on the data type of a value. For example:
var value = "Hello";
if (typeof value === "string") {
console.log("The value is a string.");
} else if (typeof value === "number") {
console.log("The value is a number.");
} else if (typeof value === "boolean") {
console.log("The value is a boolean.");
} else {
console.log("The value is of an unknown type.");
}
Handling Unknown Data Types
The `typeof` operator can be used to handle unknown or unexpected data types. By checking for specific types, you can gracefully handle different scenarios. For example:
function processValue(value) {
if (typeof value === "string") {
// Handle string values
} else if (typeof value === "number") {
// Handle number values
} else {
// Handle unknown types
}
}
0 Comments