JavaScript Functions
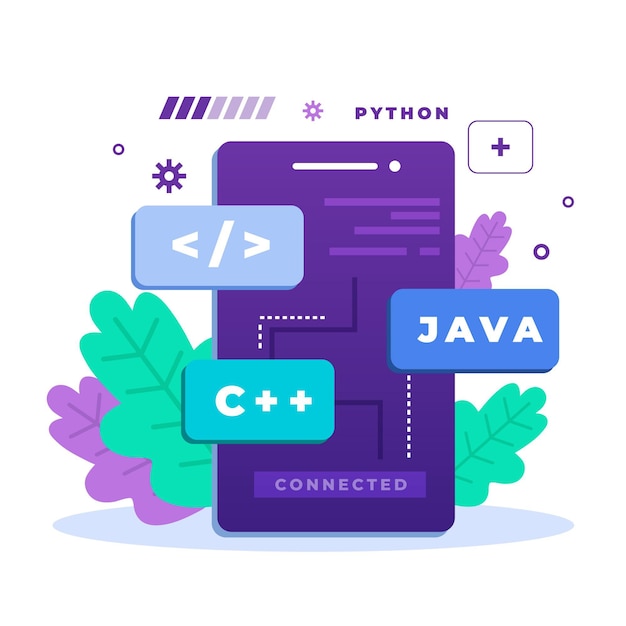
Credit: Image by Freepik
JavaScript functions are reusable blocks of code that perform specific tasks. They allow you to organize and encapsulate functionality, making your code more modular and easier to maintain. Here are a few examples of JavaScript functions:
Example 1: Addition
This function adds two numbers and returns the result:
<script>
function addNumbers(a, b) {
return a + b;
}
var sum = addNumbers(5, 3);
console.log(sum); // Output: 8
</script>
Example 2: String Length
This function calculates and returns the length of a string:
<script>
function calculateStringLength(str) {
return str.length;
}
var length = calculateStringLength("Hello, World!");
console.log(length); // Output: 13
</script>
Example 3: Random Number
This function generates and returns a random number between a specified range:
<script>
function getRandomNumber(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
var randomNumber = getRandomNumber(1, 10);
console.log(randomNumber); // Output: Random number between 1 and 10
</script>
Conclusion
JavaScript functions play a crucial role in writing clean and organized code. They allow you to encapsulate functionality and reuse it throughout your programs. By leveraging functions effectively, you can enhance code readability, maintainability, and modularity in your JavaScript projects.
0 Comments