JavaScript Introduction
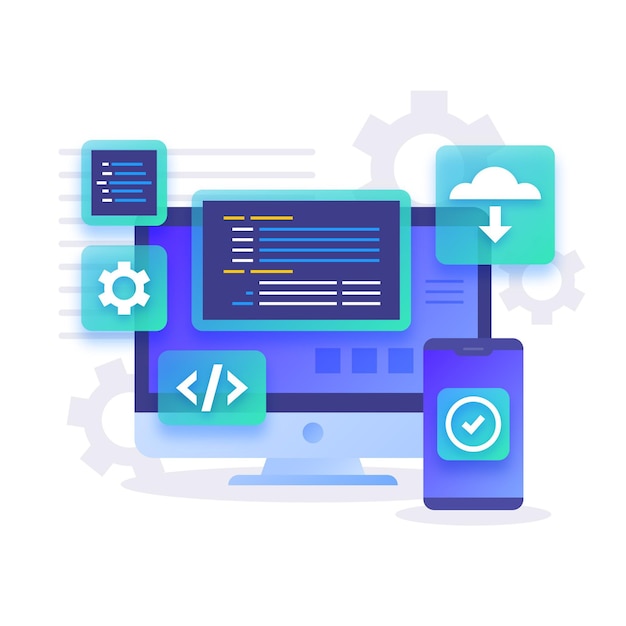
Credit: Image by Freepik
JavaScript is a popular programming language used for web development. It allows you to add interactivity and dynamic behavior to your websites.
Example: Displaying a Welcome Message
How to Include JavaScript in HTML
You can include JavaScript code in your HTML file using the <script>
tag. There are two common ways to do this:
- Inline JavaScript: You can write JavaScript code directly within the
<script>
tags in your HTML file. - External JavaScript file: You can write your JavaScript code in a separate .js file and include it in your HTML file using the
<script src="path/to/your/script.js"></script>
tag.
Conclusion
This is just a brief introduction to JavaScript. It has many more features and capabilities that make it a powerful programming language for web development. With JavaScript, you can create interactive forms, handle events, manipulate the DOM, make asynchronous requests, and much more.
Introduction to JavaScript
JavaScript is a high-level, interpreted programming language that is primarily used for adding interactivity and dynamic functionality to web pages. It was initially developed for web browsers, but now it is widely used on both the client-side and server-side of web development.
Key Features of JavaScript
- Client-Side Interactivity: JavaScript enables client-side interactivity by allowing developers to create dynamic web content. It can handle events, respond to user actions, and modify the content and behavior of web pages in real-time.
- Wide Browser Support: JavaScript is supported by all modern web browsers, making it a versatile language for building web applications that can run on different platforms.
- Object-Oriented: JavaScript is an object-oriented language, which means it organizes code into reusable objects that have properties and methods.
- Integration with HTML and CSS: JavaScript seamlessly integrates with HTML and CSS, allowing developers to manipulate the Document Object Model (DOM) and style web pages dynamically.
- Server-Side Development: With the introduction of server-side JavaScript frameworks like Node.js, JavaScript is now used for server-side development as well. It allows developers to build scalable and efficient web applications.
Example: Hello, World!
Let's start with a classic example of displaying "Hello, World!" using JavaScript:
<html>
<head>
<title>Hello, World!</title>
<script>
document.write("Hello, World!");
</script>
</head>
<body>
...
</body>
</html>
The JavaScript code in the example uses the `document.write()` method to output the "Hello, World!" message to the web page.
Conclusion
JavaScript is a powerful and versatile programming language that enhances the interactivity and functionality of web pages. With its widespread adoption and continuous evolution, it has become an essential tool for web developers.
JavaScript Fundamentals
JavaScript is a dynamic scripting language that offers a wide range of features to build interactive web applications. In this article, we will explore some fundamental concepts of JavaScript.
Data Types and Variables
JavaScript has several built-in data types, including numbers, strings, booleans, arrays, objects, and more. Variables are used to store and manipulate data. To declare a variable, you can use the `var`, `let`, or `const` keyword. For example:
<script>
var message = "Hello, World!";
let count = 10;
const PI = 3.14;
</script>
Control Flow and Functions
JavaScript provides control flow statements like conditional statements (`if`, `else if`, `else`), loops (`for`, `while`), and switch statements for making decisions and repeating actions. Functions are reusable blocks of code that perform a specific task. Here's an example of a function that adds two numbers:
<script>
function addNumbers(a, b) {
return a + b;
}
var result = addNumbers(5, 3);
console.log(result); // Output: 8
</script>
DOM Manipulation and Event Handling
JavaScript can interact with the Document Object Model (DOM) to manipulate web page elements dynamically. You can access and modify HTML elements, change their styles, handle events like button clicks, form submissions, and more. Here's a simple example that changes the text color on button click:
<html>
<body>
<button id="myButton" onclick="changeColor()">Click Me</button>
<script>
function changeColor() {
var button = document.getElementById("myButton");
button.style.color = "red";
}
</script>
</body>
</html>
Conclusion
JavaScript provides a robust foundation for building dynamic and interactive web applications. By mastering JavaScript fundamentals, you'll be equipped with the knowledge to create powerful and engaging web experiences.
0 Comments