Introduction to JavaScript Objects
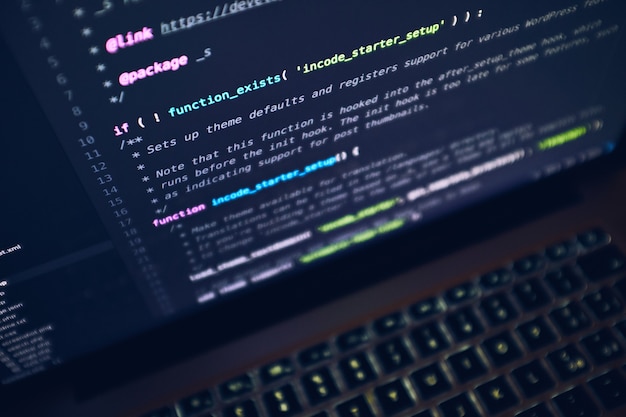
In JavaScript, an object is a collection of key-value pairs, where each key is a string (also known as a property) and each value can be any data type, including other objects. Objects provide a powerful way to represent complex entities and organize related data and functionality. In this article, we will introduce JavaScript objects and provide examples to illustrate their usage.
Creating an Object
There are different ways to create objects in JavaScript. One way is to use object literal notation, which uses curly braces ({}) to define the object and its properties. For example:
var person = {
name: 'John Doe',
age: 25,
isStudent: true
};
Accessing Object Properties
Object properties can be accessed using dot notation or bracket notation. Dot notation is used when the property name is known and is a valid identifier. For example:
console.log(person.name); // Output: "John Doe"
Bracket notation is used when the property name is stored in a variable or is not a valid identifier. For example:
var propertyName = 'age';
console.log(person[propertyName]); // Output: 25
Modifying Object Properties
Object properties can be modified by assigning a new value to them. For example:
person.age = 30;
console.log(person.age); // Output: 30
Conclusion
JavaScript objects are a fundamental concept in the language, allowing you to represent complex entities and organize related data and functionality. By understanding how to create, access, and modify object properties, you can leverage the power of objects in your JavaScript code. Experiment with objects in your own code to deepen your understanding and explore their capabilities further.
Working with JavaScript Object Methods
In JavaScript, object methods are functions that are stored as object properties. They provide a way to encapsulate logic and behavior within an object. Object methods can access and manipulate the object's properties, as well as perform other tasks. In this article, we will explore JavaScript object methods and provide examples to illustrate their usage.
Defining Object Methods
Object methods are defined as function expressions or function declarations within an object. For example:
var person = {
name: 'John Doe',
age: 25,
greet: function() {
console.log('Hello, my name is ' + this.name);
}
};
Calling Object Methods
Object methods are called using dot notation, followed by parentheses. For example:
person.greet(); // Output: "Hello, my name is John Doe"
The "this" Keyword
Inside an object method, the this
keyword refers to the object that the method belongs to. It allows the method to access and manipulate the object's properties. For example:
var person = {
name: 'John Doe',
age: 25,
greet: function() {
console.log('Hello, my name is ' + this.name + ' and I am ' + this.age + ' years old.');
}
};
person.greet(); // Output: "Hello, my name is John Doe and I am 25 years old."
Conclusion
JavaScript object methods provide a way to encapsulate logic and behavior within an object. By defining and calling object methods, you can add functionality to your objects and make them more dynamic. Understanding how to work with object methods is essential for building robust JavaScript applications. Experiment with object methods in your own code to deepen your understanding and explore their capabilities further.
JavaScript Object Constructors and Prototypes
In JavaScript, object constructors and prototypes provide a way to create and define object types and their properties and methods. Object constructors are functions used to create instances of objects, while prototypes allow objects to inherit properties and methods from other objects. In this article, we will explore JavaScript object constructors and prototypes and provide examples to illustrate their usage.
Object Constructors
Object constructors are functions that are used to create instances of objects. They serve as blueprints for creating objects with similar properties and methods. For example:
function Person(name, age) {
this.name = name;
this.age = age;
this.greet = function() {
console.log('Hello, my name is ' + this.name + ' and I am ' + this.age + ' years old.');
};
}
var person1 = new Person('John Doe', 25);
var person2 = new Person('Jane Smith', 30);
Prototypes
Prototypes allow objects to inherit properties and methods from other objects. They provide a mechanism for sharing common functionality between objects. For example:
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.greet = function() {
console.log('Hello, my name is ' + this.name + ' and I am ' + this.age + ' years old.');
};
var person = new Person('John Doe', 25);
person.greet(); // Output: "Hello, my name is John Doe and I am 25 years old."
Conclusion
JavaScript object constructors and prototypes provide a way to create and define object types and enable inheritance between objects. By using object constructors and prototypes, you can create reusable object types and share common functionality across multiple objects. Understanding how to work with constructors and prototypes is essential for building scalable and maintainable JavaScript applications. Experiment with object constructors and prototypes in your own code to deepen your understanding and explore their capabilities further.
JavaScript Object Methods and the "this" Keyword
In JavaScript, object methods are functions that are stored as object properties. They can access and manipulate the object's properties and provide behavior specific to the object. The "this" keyword is used inside object methods to refer to the object that the method belongs to. In this article, we will explore JavaScript object methods and the usage of the "this" keyword with examples.
Defining Object Methods
Object methods are defined as functions within an object. They can access the object's properties using the "this" keyword. For example:
var person = {
name: 'John Doe',
age: 25,
greet: function() {
console.log('Hello, my name is ' + this.name);
}
};
Calling Object Methods
Object methods are called using dot notation, followed by parentheses. For example:
person.greet(); // Output: "Hello, my name is John Doe"
The "this" Keyword
Inside an object method, the "this" keyword refers to the object that the method belongs to. It allows the method to access and manipulate the object's properties. For example:
var person = {
name: 'John Doe',
age: 25,
greet: function() {
console.log('Hello, my name is ' + this.name + ' and I am ' + this.age + ' years old.');
}
};
person.greet(); // Output: "Hello, my name is John Doe and I am 25 years old."
Conclusion
JavaScript object methods provide a way to encapsulate behavior within objects. By using the "this" keyword, object methods can access and manipulate the object's properties. Understanding how to define and call object methods, as well as correctly use the "this" keyword, is crucial for working with objects in JavaScript. Experiment with object methods and the "this" keyword in your own code to deepen your understanding and explore their capabilities further.
0 Comments