Addition Operator (+) in JavaScript
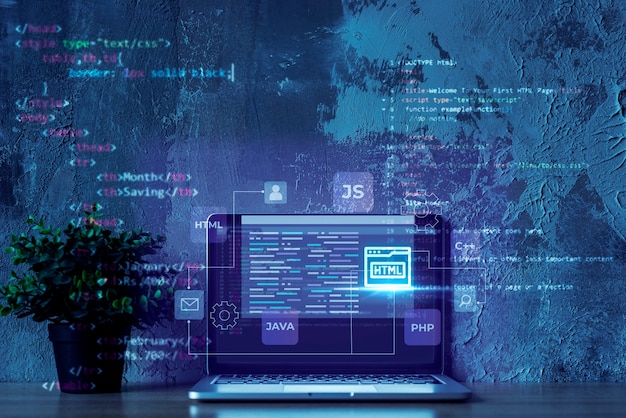
The addition operator (+
) in JavaScript is used to perform addition between numeric values or concatenate strings. In this article, we will explore the usage of the addition operator in JavaScript with various examples.
Addition of Numbers
When the addition operator is used with numeric values, it performs arithmetic addition. For example:
var x = 5;
var y = 10;
var sum = x + y;
console.log(sum); // Output: 15
Concatenation of Strings
The addition operator can also concatenate strings. When used with strings, it joins the strings together. For example:
var firstName = "John";
var lastName = "Doe";
var fullName = firstName + " " + lastName;
console.log(fullName); // Output: John Doe
Number-to-String Conversion
If one of the operands is a string, JavaScript performs automatic type conversion. It converts the number operand to a string and concatenates the values. For example:
var x = 5;
var message = "The value of x is: " + x;
console.log(message); // Output: The value of x is: 5
Conclusion
The addition operator in JavaScript can be used to perform arithmetic addition between numeric values or concatenate strings. Understanding how the addition operator behaves with different data types is crucial for writing correct and efficient JavaScript code. Experiment with the addition operator in your own code to gain a deeper understanding of its functionality.
Subtraction Operator (-) in JavaScript
The subtraction operator (-
) in JavaScript is used to perform arithmetic subtraction between numeric values. In this article, we will explore the usage of the subtraction operator in JavaScript with various examples.
Subtraction of Numbers
When the subtraction operator is used with numeric values, it performs arithmetic subtraction. For example:
var x = 10;
var y = 5;
var difference = x - y;
console.log(difference); // Output: 5
Using Negative Values
The subtraction operator can also be used with negative values. For example:
var x = 10;
var y = -5;
var result = x - y;
console.log(result); // Output: 15
Conclusion
The subtraction operator in JavaScript is used to perform arithmetic subtraction between numeric values. Understanding how the subtraction operator behaves in different scenarios is essential for accurate mathematical calculations in your JavaScript code. Experiment with the subtraction operator in your own code to explore its functionality further.
Multiplication Operator (*) in JavaScript
The multiplication operator (*
) in JavaScript is used to perform arithmetic multiplication between numeric values. In this article, we will explore the usage of the multiplication operator in JavaScript with various examples.
Multiplication of Numbers
When the multiplication operator is used with numeric values, it performs arithmetic multiplication. For example:
var x = 5;
var y = 3;
var product = x * y;
console.log(product); // Output: 15
Multiplication with Decimal Numbers
The multiplication operator can also be used with decimal numbers. For example:
var x = 2.5;
var y = 1.5;
var result = x * y;
console.log(result); // Output: 3.75
Conclusion
The multiplication operator in JavaScript is used to perform arithmetic multiplication between numeric values. Understanding how the multiplication operator behaves with different types of numbers is crucial for accurate mathematical calculations in your JavaScript code. Experiment with the multiplication operator in your own code to explore its functionality further.
Division Operator (/) in JavaScript
The division operator (/
) in JavaScript is used to perform arithmetic division between numeric values. In this article, we will explore the usage of the division operator in JavaScript with various examples.
Division of Numbers
When the division operator is used with numeric values, it performs arithmetic division. For example:
var x = 10;
var y = 2;
var result = x / y;
console.log(result); // Output: 5
Division with Decimal Numbers
The division operator can also be used with decimal numbers. For example:
var x = 7.5;
var y = 2.5;
var quotient = x / y;
console.log(quotient); // Output: 3
Conclusion
The division operator in JavaScript is used to perform arithmetic division between numeric values. Understanding how the division operator behaves with different types of numbers is crucial for accurate mathematical calculations in your JavaScript code. Experiment with the division operator in your own code to explore its functionality further.
Modulus Operator (%) in JavaScript
The modulus operator (%
) in JavaScript is used to find the remainder of a division operation between numeric values. In this article, we will explore the usage of the modulus operator in JavaScript with various examples.
Modulus of Numbers
When the modulus operator is used with numeric values, it returns the remainder of the division operation. For example:
var x = 10;
var y = 3;
var remainder = x % y;
console.log(remainder); // Output: 1
Modulus with Decimal Numbers
The modulus operator can also be used with decimal numbers. For example:
var x = 7.5;
var y = 2.5;
var result = x % y;
console.log(result); // Output: 0
Conclusion
The modulus operator in JavaScript is used to find the remainder of a division operation between numeric values. Understanding how the modulus operator behaves with different types of numbers is essential for performing calculations involving remainders in your JavaScript code. Experiment with the modulus operator in your own code to explore its functionality further.
0 Comments