Introduction to JavaScript Arrow Functions
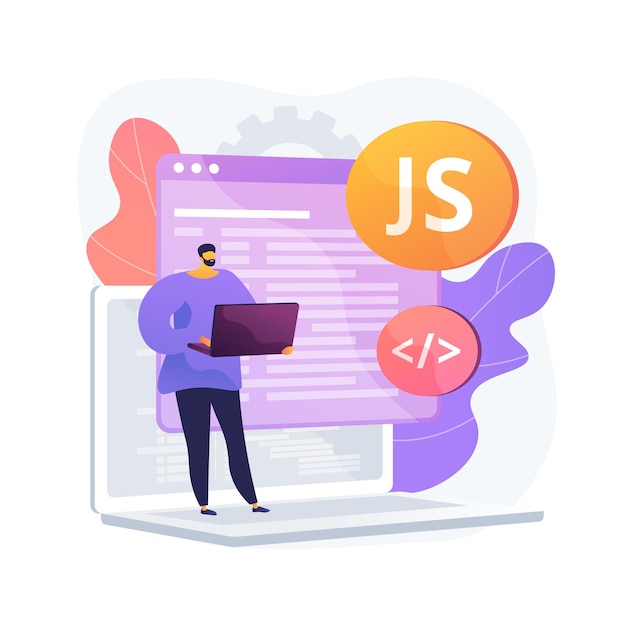
Credit: Image by vectorjuice on Freepik
Arrow functions are a concise syntax introduced in ECMAScript 6 (ES6) for defining functions in JavaScript. They provide a more concise and expressive way to write functions compared to traditional function expressions. In this article, we will introduce JavaScript arrow functions, explain their syntax, and discuss their benefits.
Syntax of Arrow Functions
Arrow functions have a simplified syntax compared to traditional function expressions. Here is an example of an arrow function:
// Traditional Function Expression
var add = function(a, b) {
return a + b;
};
// Arrow Function
var add = (a, b) => a + b;
Arrow Functions and Lexical this Binding
One of the notable features of arrow functions is their lexical this binding. Unlike traditional functions, arrow functions do not have their own this
value. Instead, they inherit the this
value from the surrounding scope. In this article, we will explore the concept of lexical this binding in arrow functions and discuss its benefits.
Example:
Consider the following example that demonstrates the lexical this binding in arrow functions:
// Traditional Function Expression
var person = {
name: "John",
sayHello: function() {
console.log("Hello, " + this.name);
}
};
// Arrow Function
var person = {
name: "John",
sayHello: () => {
console.log("Hello, " + this.name);
}
};
person.sayHello(); // Output: Hello, undefined
Benefits of Arrow Functions in JavaScript
Arrow functions bring several benefits to JavaScript code, making it more concise and readable. They simplify the syntax and provide a shorter and cleaner way to write functions. In this article, we will discuss the benefits of using arrow functions in JavaScript.
Benefits of Arrow Functions
- Shorter and more concise syntax
- Implicit return for one-line expressions
- Lexical this binding
- No binding of
this
,arguments
, andsuper
- No need for
function
keyword - Easy integration with array methods
Using Arrow Functions in JavaScript
Arrow functions can be used in various scenarios in JavaScript to simplify and enhance code readability. They are commonly used as anonymous functions, as well as in array methods and callbacks. In this article, we will explore different use cases of arrow functions in JavaScript.
Example: Using Arrow Functions as Array Callbacks
Arrow functions are often used as callbacks in array methods like map
, filter
, and reduce
. Here is an example of using an arrow function with the map
method:
var numbers = [1, 2, 3, 4, 5];
var doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
0 Comments