Introduction to JavaScript Iterables
In JavaScript, an iterable is an object that can be iterated over using a loop or other iteration mechanisms. It provides a way to access its elements in a sequential manner. Iterables are used in various JavaScript features, such as the for-of
loop and the Spread
operator. In this article, we will introduce the concept of iterables in JavaScript and provide examples to illustrate their usage.
Iterable Objects in JavaScript
JavaScript provides built-in iterable objects, such as arrays and strings. Additionally, user-defined objects can be made iterable by implementing the iterable protocol. Iterable objects must have a special method called Symbol.iterator
, which returns an iterator object. The iterator object is responsible for defining how the iteration over the elements will be done.
Example:
Consider the following example that demonstrates iteration over an array using a for-of
loop:
var fruits = ["apple", "banana", "orange"];
for (var fruit of fruits) {
console.log(fruit);
}
Creating Custom Iterables in JavaScript
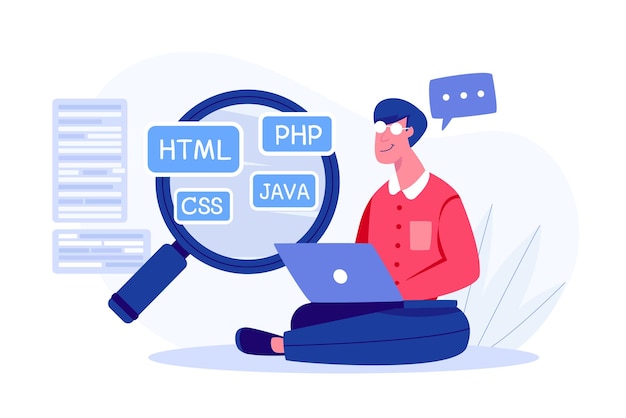
In JavaScript, you can create custom iterable objects by implementing the iterable protocol. This allows you to define your own iteration behavior for objects that are not inherently iterable, such as user-defined classes. In this article, we will explore the process of creating custom iterables in JavaScript with examples.
Iterable Protocol
To make an object iterable, you need to define a method named Symbol.iterator
on the object. This method should return an iterator object. The iterator object must have a next()
method that returns an object with value
and done
properties. The value
property represents the current element, and the done
property indicates if the iteration is complete.
Example:
Consider the following example that demonstrates the creation of a custom iterable object:
var range = {
start: 1,
end: 5,
[Symbol.iterator]: function() {
var current = this.start;
var end = this.end;
return {
next: function() {
if (current <= end) {
return { value: current++, done: false };
} else {
return { done: true };
}
}
};
}
};
for (var number of range) {
console.log(number);
}
The Iterable and Iterator Interfaces
In JavaScript, the Iterable and Iterator interfaces define the protocol for iteration over iterable objects. The Iterable interface represents objects that can be iterated, and the Iterator interface represents the iterator object responsible for the actual iteration. Understanding these interfaces is crucial for working with iterables in JavaScript. In this article, we will explore the Iterable and Iterator interfaces in detail and provide examples to illustrate their usage.
The Iterable Interface
The Iterable interface is used to define iterable objects in JavaScript. An iterable object must implement the Symbol.iterator
method, which returns the iterator object. This allows the object to be iterated using a for-of
loop or other iteration mechanisms.
The Iterator Interface
The Iterator interface is used to define iterator objects in JavaScript. An iterator object must implement the next()
method, which returns an object with the value
and done
properties. The value
property represents the current element, and the done
property indicates if the iteration is complete.
Example:
Consider the following example that demonstrates the usage of the Iterable and Iterator interfaces:
var numbers = [1, 2, 3];
var iterator = numbers[Symbol.iterator]();
console.log(iterator.next()); // { value: 1, done: false }
console.log(iterator.next()); // { value: 2, done: false }
console.log(iterator.next()); // { value: 3, done: false }
console.log(iterator.next()); // { done: true }
Working with Built-in Iterables in JavaScript
JavaScript provides several built-in iterables, such as arrays, strings, maps, and sets. These iterables can be used with the for-of
loop or other iteration mechanisms to access and process their elements. In this article, we will explore the usage of built-in iterables in JavaScript with examples.
Example:
Consider the following example that demonstrates iteration over a string using a for-of
loop:
var message = "Hello";
for (var char of message) {
console.log(char);
}
0 Comments