Assignment Operator (=) in JavaScript
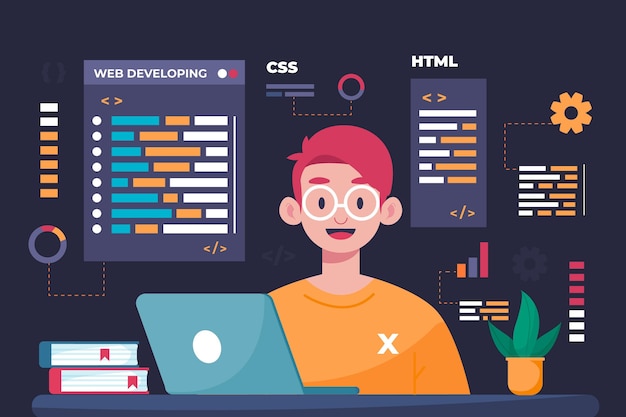
The assignment operator (=
) in JavaScript is used to assign a value to a variable. In this article, we will explore the usage of the assignment operator in JavaScript with various examples.
Assigning a Value to a Variable
The assignment operator is used to assign a value to a variable. For example:
var x;
x = 10;
console.log(x); // Output: 10
Assigning the Value of One Variable to Another
The assignment operator can also be used to assign the value of one variable to another. For example:
var x = 10;
var y;
y = x;
console.log(y); // Output: 10
Updating the Value of a Variable
The assignment operator can be used to update the value of a variable. For example:
var x = 10;
x = x + 5;
console.log(x); // Output: 15
Addition Assignment Operator (+=) in JavaScript
The addition assignment operator (+=
) in JavaScript is used to add a value to an existing variable and assign the result back to the variable. In this article, we will explore the usage of the addition assignment operator in JavaScript with various examples.
Adding a Value to an Existing Variable
The addition assignment operator adds a value to an existing variable and assigns the result back to the variable. For example:
var x = 10;
x += 5; // Equivalent to x = x + 5
console.log(x); // Output: 15
Concatenating a String to an Existing Variable
The addition assignment operator can also be used to concatenate a string to an existing variable. For example:
var message = "Hello";
message += " World"; // Equivalent to message = message + " World"
console.log(message); // Output: Hello World
Subtraction Assignment Operator (-=) in JavaScript
The subtraction assignment operator (-=
) in JavaScript is used to subtract a value from an existing variable and assign the result back to the variable. In this article, we will explore the usage of the subtraction assignment operator in JavaScript with various examples.
Subtracting a Value from an Existing Variable
The subtraction assignment operator subtracts a value from an existing variable and assigns the result back to the variable. For example:
var x = 10;
x -= 5; // Equivalent to x = x - 5
console.log(x); // Output: 5
Multiplication Assignment Operator (*=) in JavaScript
The multiplication assignment operator (*=
) in JavaScript is used to multiply an existing variable by a value and assign the result back to the variable. In this article, we will explore the usage of the multiplication assignment operator in JavaScript with various examples.
Multiplying an Existing Variable by a Value
The multiplication assignment operator multiplies an existing variable by a value and assigns the result back to the variable. For example:
var x = 5;
x *= 3; // Equivalent to x = x * 3
console.log(x); // Output: 15
0 Comments